Since this is an alarm, There's pretty much a lot of purpose in using it. It really depends totally on how you decide to implement or place it at. For my case, I'm using it as an incoming person detector. The alarm simply goes off when someone is coming into my room or when the door gets close or open. Let's get started!
Components required:
1) Arduino Uno (along with its cable)
2) USB Port Type B. ( You can change this, If you prefer to use USB Type A, go ahead)
3) Some jumper wires
4) 5V Magnetic Buzzer
5) LED (Any colour, I chose red)
6) PCB Slide Switch
7) Attiny 85
8) 8 Pin IC Holder
9) Strip Board
10) Female Pin Header ( I used the snap-able type. I find it easier to use that)
11) Ultrasonic Sensor (Parallax ping sensor)
Tools required:
1) Solder wire and tool
2) Wire Cutter
Step 1)
The pinout for AtTiny 85 are as follow. Do take note that pin 1 and 8 are the side nearest to the notch or dot.
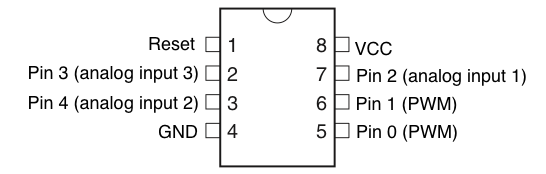
Pin 1-3 of the chip will not be connected.
Pin 4 of the chip will be the ground, It will be connected to the ground from the power source, led, buzzer and ultrasonic sensor.
Pin 5 of the chip will be connected to the Anode of the Led. The Cathode of the Led will be connected to the anode of the buzzer. The cathode of the buzzer will be connected to the ground. This way, the buzzer will buzz when the Led turns on.
Pin 6 of the chip will be connected to the UltraSonic Sensor (Sensor Pin).
Pin 7 of the chip will not be connected.
Pin 8 of the chip will be the Vcc or where the Vcc from your Type B USB Port will be going to. A switch should be placed between this pin and the Vcc from the USB Port.
Step 2)
Break at the necassary places to avoid the circuit from crossing each other. Mine consists of 7 break point.
After all connections is done, you should have something like this. Note, this is my layout, yours may be different. As long as the components are connected according to the steps above, it should be working fine.
Step 3)
Install your arduino as ISP . Refer to my previous post. The AtTiny can now be programmed with the shield and Arduino. Copy the code below to your Arduino IDE and upload to your AtTiny. Proper steps for downloading to the AtTiny can be found in my previous post.
Copy code from below this line onwards:
const int pingPin = 1;
void setup() {
pinMode(0, OUTPUT); //Led
}
void loop()
{
long duration, inches, cm;
pinMode(pingPin, OUTPUT);
digitalWrite(pingPin, LOW);
delayMicroseconds(2);
digitalWrite(pingPin, HIGH);
delayMicroseconds(5);
digitalWrite(pingPin, LOW);
pinMode(pingPin, INPUT);
duration = pulseIn(pingPin, HIGH);
// convert the time into a distance
inches = microsecondsToInches(duration);
cm = microsecondsToCentimeters(duration);
delay(5);
if(cm<50 && cm>20)
{
digitalWrite(0, HIGH); // turn the LED on (HIGH is the voltage level)
delay(200); // wait for a second
digitalWrite(0, LOW); // turn the LED off by making the voltage LOW
delay(200); // wait for a second
}
else if(cm<20)
{
digitalWrite(0, HIGH); // turn the LED on (HIGH is the voltage level)
delay(80); // wait for a second
digitalWrite(0, LOW); // turn the LED off by making the voltage LOW
delay(80); // wait for a second
}
}
long microsecondsToInches(long microseconds)
{
return microseconds / 74 / 2;
}
long microsecondsToCentimeters(long microseconds)
{
return microseconds / 29 / 2;
}
Copy code till above this line
Upload the code to your AtTiny 85.
Step 4)
Your board shall now work as follows: I've not connected the buzzer in the video.
Step 5)
As mine is an incoming people sensor. I'm placing it near my room door.
This is the final outcome. My very own alarm that alerts me when someone is approaching or entering the room.
Hope you'll give this project a try. Cheers!